Photos have develop into among the used types of content material materials in fashionable web functions. Although using background pictures improves the making use of’s really feel and seem, rising image sizes can significantly have an effect on utility effectivity.
Even when appropriately optimized, pictures can weigh pretty a bit and protect prospects able to entry content material materials in your web page. Usually, they get impatient and navigate some place else besides you provide you with a solution to image loading that doesn’t intrude with the notion of tempo.
On this text, you’ll research 5 approaches to lazy loading pictures which you’ll add to your web optimization toolkit to reinforce the individual experience in your web page. You need to use these methods to lazy load quite a few image varieties in your utility along with background pictures, inline pictures, and banner pictures.
Key Takeaways
- Lazy loading pictures can improve web page effectivity by loading pictures asynchronously, solely after the above-the-fold content material materials is completely loaded, or solely after they appear inside the browser’s viewport. This might make a distinction in individual experience, notably for these accessing the Internet on cell devices and slow-connections.
- Native lazy loading is a straightforward methodology using HTML that has zero overhead, nevertheless not all browsers help this attribute. The Intersection Observer API is one different methodology that is simple to implement and environment friendly, nevertheless as soon as extra, not all browsers help this.
- JavaScript libraries equal to Lozad.js and Yall.js might be utilized for quick and simple implementation of lazy loading of pictures, films, iframes and additional. They use the Intersection Observer API, nevertheless browser help have to be thought-about.
- An fascinating affect could possibly be achieved with lazy loading by way of using a blurred, low-resolution copy of the image whereas the high-res mannequin is being lazy loaded, as seen on Medium. This can be achieved in quite a few strategies, such as a result of the tactic by Craig Buckler, which may be very performant and helps retina screens.
What Is Lazy Loading?
Lazy loading pictures means loading pictures on internet sites asynchronously. You probably can lazy load content material materials on scroll after the above-the-fold content material materials is completely loaded or conditionally when it solely needs to look inside the browser’s viewport. Which signifies that if prospects don’t scroll all the way in which wherein down, pictures positioned on the bottom of the online web page obtained’t even be loaded, in the long run enhancing the making use of effectivity.
Understanding one of the best ways to permit lazy loading in HTML is essential for web builders as most internet sites require this methodology. As an example, attempt wanting your favorite on-line wanting ground for high-res photos, and in addition you’ll rapidly perceive that the web page lots solely a restricted number of pictures. As you scroll down the online web page, the placeholder image quickly fills up with exact image for preview.
For instance, uncover the loader on Unsplash.com: scrolling that portion of the online web page into view triggers the substitute of a placeholder with a full-res {photograph}:
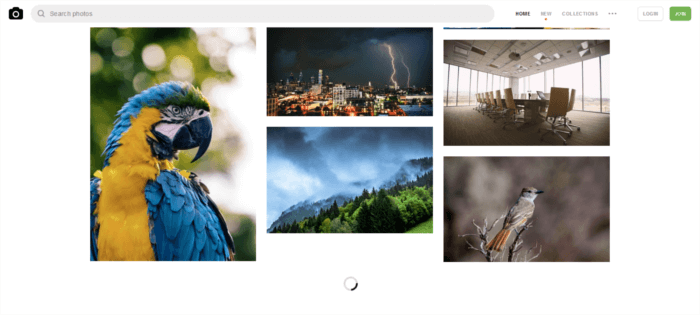
Why You Should Implement Lazy Loading for Photos?
Understanding one of the best ways to lazy load pictures is important for optimizing web effectivity, notably on pages with heavy seen content material materials. Listed beneath are a couple of wonderful the rationale why it is best to have in mind lazy loading pictures in your web page:
1. Improves DOM Loading Time
- In case your web page makes use of JavaScript to point out content material materials or current some type of efficiency to prospects, loading the DOM quickly turns into important. It’s widespread for scripts to attend until the DOM has completely loaded sooner than they start working. On a web page with a serious number of pictures, lazy loading — or loading pictures asynchronously — could make the excellence between prospects staying or leaving your web page.
2. Restricted Bandwith Use
- Since most lazy loading choices work by loading pictures offered that the individual scrolls to the scenario the place pictures could possibly be seen contained within the viewport, these pictures will not ever be loaded if prospects on no account get to that point. This suggests considerable bandwidth monetary financial savings, for which most prospects, notably these accessing the Internet on cell devices and gradual connections, might be thanking you.
Properly, lazy loading pictures help with web page effectivity, nevertheless what’s the best option to do it?
There’s no glorious means.
If you happen to occur to remain and breathe JavaScript, implementing your private lazy loading reply shouldn’t be a problem. Nothing provides you additional administration than coding one factor your self.
Alternatively, you probably can browse the Internet for viable approaches or be a part of a dialogue Dialogue board and share ideas. I did merely that and obtained right here all through these 5 fascinating methods.
1. Native Lazy Loading
Native lazy loading of pictures and iframes is a straightforward methodology to lazy load content material materials when an individual scrolls the net internet web page. You merely need in order so as to add the loading=”lazy” attribute to your pictures and iframes.

As you probably can see, no JavaScript, no dynamic swapping of the src attribute’s price, merely plain outdated HTML. This system is a perfect occasion of one of the best ways so as to add lazy loading in HTML with none additional overhead.
The “loading” attribute provides us the selection to delay off-screen pictures and iframes until prospects scroll to their location on the internet web page. loading can take any of these three values:
- lazy: Works good for lazy loading
- eager: Instructs the browser to load the specified content material materials instantly
- auto: Leaves the selection to lazy load or to not lazy load as a lot because the browser.
This system has no rivals: it has zero overhead, and it’s a transparent and simple methodology to lazy load pictures in HTML. However, although most important browsers have good help for the “loading” attribute, some browsers nonetheless lack full help as of the time of writing.
For an in-depth article on this superior attribute for HTML lazy load pictures, along with browser help workarounds, don’t miss Addy Osmani’s “Native image lazy-loading for the net!“.
2. Lazy Loading Using the Intersection Observer API
The Intersection Observer API is a up to date interface which you’ll leverage for lazy loading pictures and totally different content material materials.
Proper right here’s how MDN introduces this API:
The Intersection Observer API provides a technique to asynchronously observe modifications inside the intersection of a purpose ingredient with an ancestor ingredient or with a top-level doc’s viewport.
In numerous phrases, Intersection Observer API watches the intersection of 1 ingredient with one different asynchronously.
Denys Mishunov has an essential tutorial every on the Intersection Observer and on lazy loading pictures using it. Proper right here’s what his reply looks like.
Let’s say you’d favor to lazy load an image gallery. The markup for each image would look like this:
![test image]()
Proper right here, the path to the image is contained inside a data-src attribute, not a src attribute. The reason is that using src means the image would load instantly, which is not what you want.
Throughout the CSS, it’s possible you’ll give each image a min-height price, let’s say 100px. This provides each image placeholder (the img ingredient with out the src attribute) a vertical dimension:
img {
} min-height: 100px;
/* additional varieties proper right here */
Then, inside the JavaScript doc, it’s advisable create a config object and register it with an intersectionObserver event:
// create config object: rootMargin and threshold
// are two properties uncovered by the interface
const config = {
rootMargin: '0px 0px 50px 0px',
threshold: 0
};
// register the config object with an event
// of intersectionObserver
let observer = new intersectionObserver(function(entries, self) {
// iterate over each entry
entries.forEach(entry => {
// course of merely the pictures which may be intersecting.
// isIntersecting is a property uncovered by the interface
if(entry.isIntersecting) {
// personalized function that copies the path to the img
// from data-src to src
preloadImage(entry.purpose);
// the image is now in place, stop watching
self.unobserve(entry.purpose);
}
});
}, config);
Lastly, you probably can iterate over your entire pictures and add them to this iterationObserver event:
const imgs = doc.querySelectorAll('[data-src]');
imgs.forEach(img => {
observer.observe(img);
});
The deserves of this reply are that it’s a breeze to implement, it’s environment friendly, and it has the intersection. Observer does the heavy lifting by the use of calculations.
With reference to browser help, the entire important browsers help Intersection Observer API of their latest variations, apart from IE 11 and Opera Mini.
You probably can research additional regarding the Intersection Observer API and the details of this implementation in Denys’s article.
3. Lozad.js
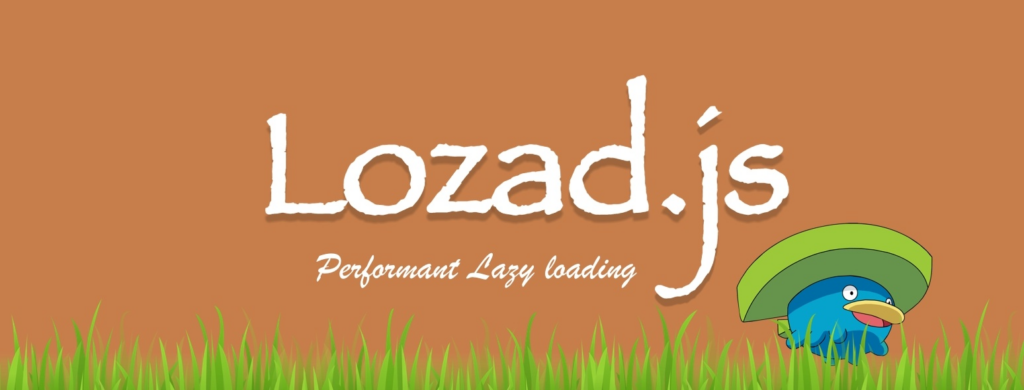
A quick and simple totally different for implementing lazy loading of pictures is to let a JS library do a number of the job for you.
Lozad.js is a extraordinarily performant, light, and configurable lazy loader that makes use of pure JavaScript with no dependencies. It’s an excellent instrument for lazy loading JavaScript when an individual scrolls pictures, films, and iframes.
You probably can arrange Lozad with npm/Yarn and import it using your module bundler of choice:
npm arrange --save lozad
yarn add lozad
import lozad from 'lozad';
Alternatively, you probably can merely get hold of the library using a CDN and add it to the underside of the HTML internet web page in a tag:
Next, for a basic implementation, add the class lozad to the asset in your markup:
![]()
Finally, instantiate Lozad in your JS document:
const observer = lozad();
observer.observe();
You’ll find all the details of using the library on the Lozad GitHub repository.
If you don’t want to learn about the Intersection Observer API or you’re simply looking for a fast implementation that applies to a variety of content types, Lozad is a great choice.
4. Lazy Loading with Blurred Image Effect
If you’re a Medium reader, you have certainly noticed how the site loads the main image inside a post. The first thing you see is a blurred, low-resolution copy of the image, while its high-res version is being lazy loaded:
You can achieve a similar effect when you lazy load images in HTML by using CSS and JavaScript together.
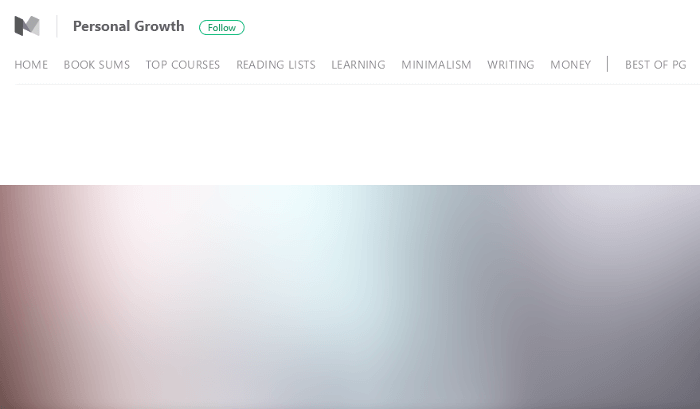
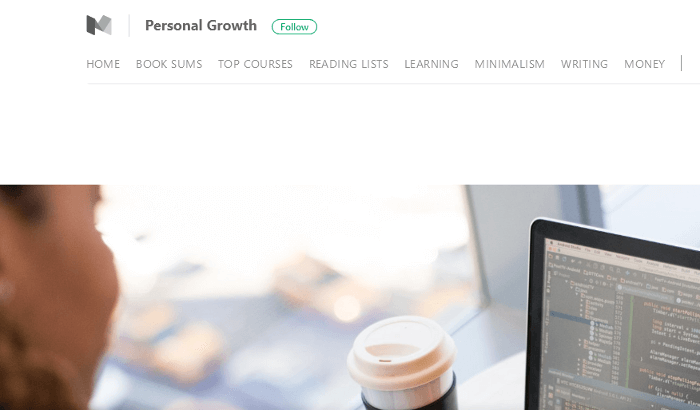
You can add lazy load to images with this interesting blurring effect in a number of ways.
My favorite technique is by Craig Buckler. Here’s all the goodness of this solution:
- Performance: Only 463 bytes of CSS and 1,007 bytes of minified JavaScript code.
- Support for retina screens.
- Dependency-free: No jQuery or other libraries and frameworks are required.
- Progressively enhanced to counteract older browsers and failing JavaScript.
You can read all about it in How to Build Your Own Progressive Image Loader and download the code on the project’s GitHub repo.
5. Yall.js
Yall.js is a feature-packed JavaScript library that uses Intersection Observer API for lazy loading JavaScript when a user scrolls. It supports images, videos, iframes, and smartly falls back on traditional event handler techniques where necessary.
When including Yall in your document, you need to initialize it as follows:
Subsequent, to lazy load a straightforward img ingredient, all it's advisable do in your markup is:

Phrase the subsequent:
- You add the class lazy to the ingredient.
- The value of src is a placeholder image.
- The path to the image it is advisable lazy load is contained within the data-src attribute.
Listed beneath are the benefits of Yall.js:
- Good effectivity with the Intersection Observer API.
- Inconceivable browser help (it goes once more to IE11).
- No totally different dependencies are important.
To check additional about what Yall.js can present and for additional superior implementations, be at liberty to check out the enterprise’s internet web page on GitHub.
Conclusion
And there you could have it — 5 strategies of lazy loading pictures you'll be able to start to experiment with and try out in your initiatives. Understanding one of the best ways to lazy load pictures is a helpful capacity for any web developer., you probably can research additional methods or share your expertise with others by changing into a member of this SitePoint dialogue board on image optimization.
FAQs About Lazy Loading Photos
What Is Lazy Loading Photos?
Lazy loading is a web based development methodology used to reinforce the effectivity of web pages by deferring the loading of certain elements, equal to pictures until they're needed. Lazy loading pictures signifies that pictures are loaded solely after they enter the individual’s viewport or develop into seen on the web internet web page, fairly than loading all pictures as rapidly as a result of the online web page is initially rendered.
What Are the Benefits of Lazy Loading Photos?
- Improved web page effectivity
- Sooner preliminary internet web page rendering, and lowered bandwidth utilization.
- Enhance the individual experience.
- Reduce the server load and improve the overall effectivity of your web utility.
Lazy Load Photos in HTML?
In order so as to add lazy load to pictures in HTML, you need to use the “loading” attribute. The “loading” attribute is a standard HTML attribute meaning that you would be able to administration when an image have to be loaded. To permit HTML lazy load pictures, add the “loading” attribute with the value “lazy” to the img ingredient. This tells the browser to load the image solely when it is about to enter the viewport. Proper right here’s an occasion:

Is Lazy Loading Photos Good?
Positive, using lazy load pictures HTML is taken under consideration a useful comply with in web development for numerous causes. It enhances internet web page loading tempo by deferring non-essential image loading, resulting in faster preliminary internet web page rendering and improved individual experience. This methodology conserves bandwidth, making it advantageous for purchasers with restricted data plans or slower internet connections. Furthermore, it positively impacts search engine advertising (search engine optimisation) by boosting internet web page loading tempo, and it aligns with the concepts of progressive enhancement. By reducing perceived wait situations and providing seen cues all through loading, lazy loading contributes to a smoother and additional setting pleasant wanting experience, notably on cell devices.
How Do You Know If an Image Is Lazy Loaded?
To confirm for individuals who’ve precisely utilized one of the best ways to lazy load pictures HTML, you probably can study the HTML provide code or use browser developer devices. By right-clicking on the image and selecting “Look at” or “Look at Issue,” you probably can have a look at the img ingredient representing the image inside the developer devices panel. Seek for the presence of the “loading” attribute all through the img ingredient. If the “loading” attribute is able to “lazy,” it signifies that the image is configured for lazy loading. Using browser developer devices, you probably can quickly confirm the lazy loading standing of pictures by inspecting their attributes.
How Can I Implement Lazy Loading for Photos Using JavaScript?
To implement lazy loading for pictures using JavaScript, you need to use libraries like Lozad.js or Yall.js, otherwise you probably can write your private personalized reply using the Intersection Observer API. These libraries allow you to lazy load content material materials on scroll by monitoring when elements enter the viewport and loading them solely at that second.
Does Lazy Loading Photos Impact search engine optimisation?
Positive, lazy loading pictures can positively affect search engine optimisation. By enhancing internet web page load situations and reducing the amount of data that have to be loaded initially, lazy loading may assist your web page rank larger in search engine outcomes. Sooner internet web page speeds are a acknowledged score concern for search engines like google and yahoo like google and yahoo like Google, and lazy loading can contribute to this by making sure that your web page’s pictures don’t decelerate the overall effectivity.
What Is the Distinction Between Native Lazy Loading and JavaScript-Primarily based Lazy Loading?
Native lazy loading is determined by the browser’s built-in help for the “loading” attribute in and
Are There Any Downsides to Lazy Loading Photos?
The precept draw again is that pictures may load with a slight delay as a result of the individual scrolls down the online web page. Moreover, some older browsers could not help lazy loading.
Can I Lazy Load Photos in WordPress?
Positive, you probably can merely lazy load pictures in WordPress. Many themes now help native lazy loading by default, nevertheless you can also use plugins like “Lazy Load by WP Rocket” or “Smush” to implement lazy loading with none coding.