Proper right here’s how which may look:
This building is a straightforward occasion of discover ways to create quiz HTML code that serves as a foundation in your JavaScript quiz template. Within the occasion you run the equipment now, you may merely see a “Submit Quiz” button.
Step 2 – Initialize JavaScript Variables
Now, we’ll use the JavaScript doc.getElementById method to select the above HTML elements and retailer references to them throughout the JavaScript quiz code like beneath:
const quizContainer = doc.getElementById('quiz');
const resultsContainer = doc.getElementById('outcomes');
const submitButton = doc.getElementById('submit');
The next issue our quiz app needs is a number of inquiries to indicate. We’ll use JavaScript object literals to represent the particular person questions and an array to hold all the questions that make up our quiz app. Using an array will make the questions easy to iterate over:
const myQuestions = [
{
question: "Who invented JavaScript?",
answers: {
a: "Douglas Crockford",
b: "Sheryl Sandberg",
c: "Brendan Eich"
},
correctAnswer: "c"
},
{
question: "Which one of these is a JavaScript package manager?",
answers: {
a: "Node.js",
b: "TypeScript",
c: "npm"
},
correctAnswer: "c"
},
{
question: "Which tool can you use to ensure code quality?",
answers: {
a: "Angular",
b: "jQuery",
c: "RequireJS",
d: "ESLint"
},
correctAnswer: "d"
}
];
Be completely satisfied to position in as many questions or options as you want.
Observe: As that’s an array, the questions will appear throughout the order they’re listed. In the event you want to form the questions in any technique sooner than presenting them to the buyer, strive our quick tip on sorting an array of objects in JavaScript.
Step 3 – Assemble the Quiz Function
Now that now we have now our document of questions, we’ll current them on the internet web page. For that, we will be using a function named buildQuix(). Let’s bear the subsequent JavaScript line by line to see the best way it really works:
function buildQuiz(){
// variable to retailer the HTML output
const output = [];
// for each question...
myQuestions.forEach(
(currentQuestion, questionNumber) => {
// variable to retailer the document of doable options
const options = [];
// and for each accessible reply...
for(letter in currentQuestion.options){
// ...add an HTML radio button
options.push(
``
);
}
// add this question and its options to the output
output.push(
` ${currentQuestion.question}
${options.be a part of('')}
`
);
}
);
// lastly combine our output document into one string of HTML and put it on the internet web page
quizContainer.innerHTML = output.be a part of('');
}
First, we create an output variable to comprise all the HTML output, along with questions and reply picks.
Subsequent, we’ll start establishing the HTML for each question. We’ll should loop by means of each question like this:
myQuestions.forEach( (currentQuestion, questionNumber) => {
// the code we want to run for each question goes proper right here
});
For brevity, we’re using an arrow function to hold out our operations on each question. On account of that’s in a forEach loop, we get the current price, the index (the place amount of the current merchandise throughout the array), and the array itself as parameters. We solely need the current price and the index, which for our capabilities, we’ll determine currentQuestion and questionNumber respectively.
Now let’s take a look on the code inside our loop:
// we'll want to retailer the document of reply picks
const options = [];
// and for each accessible reply...
for(letter in currentQuestion.options){
// ...add an html radio button
options.push(
``
);
}
// add this question and its options to the output
output.push(
` ${currentQuestion.question}
${options.be a part of('')}
`
);
For each question, we’ll want to generate the right HTML. So, our first step is to create an array to hold the document of doable options.s.
Subsequent, we’ll use a loop to fill throughout the doable options for the current question. For each choice, we’re creating an HTML radio button, which we enclose in a
Proper right here, we’re using template literals, which are strings nonetheless further extremely efficient. We’ll make use of the subsequent choices of template literals:
- Multi-line capabilities.
- Don’t need to make use of escape quotes inside quotes on account of template literals use backticks.
- String interpolation permits embedding JavaScript expressions correct into your strings like this: ${code_goes_here}.
As quickly as now we have now our document of reply buttons, we’ll push the question HTML and the reply HTML onto our whole document of outputs.
Uncover that we’re using a template literal and some embedded expressions to first create the question div after which create the reply div. The be a part of expression takes our document of options and locations them collectively in a single string that we are going to output into our options div.
Now that we’ve generated the HTML for each question, we shall be a part of all of it collectively and current it on the internet web page:
quizContainer.innerHTML = output.be a part of('');
Now, our buildQuiz function is full, and it’s best to be able to run the quiz app and see the questions displayed.
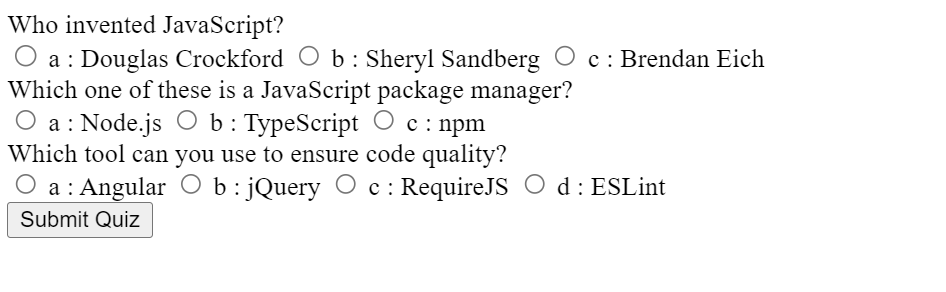
Nonetheless, the development of your code is important. On account of one factor known as the temporal lifeless zone, you might’t reference your question array sooner than it has been outlined.
To recap, that’s the correct building:
// Capabilities
function buildQuiz(){ ... }
function showResults(){ ... }
// Variables
const quizContainer = doc.getElementById('quiz');
const resultsContainer = doc.getElementById('outcomes');
const submitButton = doc.getElementById('submit');
const myQuestions = [ ... ];
// Kick points off
buildQuiz();
// Event listeners
submitButton.addEventListener('click on on', showResults);
Step 4 – Displaying the Quiz Outcomes
At this degree, we want to assemble out our showResults function to loop over the options, take a look at them, and current the outcomes. It’s a important part of any quiz recreation JavaScript implementation, as a result of it provides speedy recommendations to the buyer based totally on their effectivity.
Proper right here’s the function, which we’ll bear intimately subsequent:
function showResults(){
// accumulate reply containers from our quiz
const answerContainers = quizContainer.querySelectorAll('.options');
// protect monitor of shopper's options
let numCorrect = 0;
// for each question...
myQuestions.forEach( (currentQuestion, questionNumber) => {
// uncover chosen reply
const answerContainer = answerContainers[questionNumber];
const selector = `enter[name=question${questionNumber}]:checked`;
const userAnswer = (answerContainer.querySelector(selector) || {}).price;
// if reply is correct
if(userAnswer === currentQuestion.correctAnswer){
// add to the number of proper options
numCorrect++;
// shade the options inexperienced
answerContainers[questionNumber].kind.shade="lightgreen";
}
// if reply is inaccurate or clear
else{
// shade the options purple
answerContainers[questionNumber].kind.shade="purple";
}
});
// current number of proper options out of full
resultsContainer.innerHTML = `${numCorrect} out of ${myQuestions.measurement}`;
}
First, we select all of the reply containers in our quiz’s HTML. Then, we’ll create variables to take care of monitor of the buyer’s current reply and the general number of proper options.
// accumulate reply containers from our quiz
const answerContainers = quizContainer.querySelectorAll('.options');
// protect monitor of shopper's options
let numCorrect = 0;
Now, we’ll loop by means of each question and take a look at the options.
We will be using 3 steps for that:
- Uncover the chosen reply throughout the HTML.
- Cope with what happens if the reply is correct.
- Cope with what happens if the reply is inaccurate.
Let’s look further fastidiously at how we’re discovering the chosen reply in our HTML:
// uncover chosen reply
const answerContainer = answerContainers[questionNumber];
const selector = `enter[name=question${questionNumber}]:checked`;
const userAnswer = (answerContainer.querySelector(selector) || {}).price;
First, we’re guaranteeing we’re wanting contained in the reply container for the current question.
Throughout the subsequent line, we’re defining a CSS selector that may enable us to find which radio button is checked.
Then we’re using JavaScript’s querySelector to hunt for our CSS selector throughout the beforehand outlined answerContainer. In essence, which signifies that we’ll uncover which reply’s radio button is checked.
Lastly, we’ll get the price of that reply by using .price.
Dealing with Incomplete Client Enter
What if the buyer has left an answer clear? On this case, using .price would set off an error on account of you might’t get the price of 1 factor that’s not there. To resolve this, we’ve added ||, which suggests “or”, and {}, which is an empty object. Now, the overall assertion says:
- Get a reference to our chosen reply part OR, if that doesn’t exist, use an empty object.
- Get the price of regardless of was throughout the first assertion.
In consequence, the price will each be the buyer’s reply or undefined, which suggests a shopper can skip a question with out crashing our quiz app.
Evaluating the Options and Displaying the Consequence
The next statements in our answer-checking loop will enable us to cope with proper and incorrect options.
// if reply is correct
if(userAnswer === currentQuestion.correctAnswer){
// add to the number of proper options
numCorrect++;
// shade the options inexperienced
answerContainers[questionNumber].kind.shade="lightgreen";
}
// if reply is inaccurate or clear
else{
// shade the options purple
answerContainers[questionNumber].kind.shade="purple";
}
If the buyer’s reply matches the right choice, enhance the number of proper options by one and (optionally) shade the set of picks inexperienced. If the reply is inaccurate or clear, shade the reply picks purple (as soon as extra, elective).
As quickly because the answer-checking loop is accomplished, we’ll current what variety of questions the buyer purchased correct:
// current number of proper options out of full
resultsContainer.innerHTML = `${numCorrect} out of ${myQuestions.measurement}`;
And now now we have now a working JavaScript quiz!
Within the occasion you’d like, you might wrap all the quiz in an IIFE (immediately invoked function expression), which is a function that runs as rapidly as you define it. This may increasingly protect your variables out of world scope and ensure that your quiz app doesn’t intrude with another scripts working on the internet web page.
(function(){
// put the rest of your code proper right here
})();
Now you’re all set! Be completely satisfied in order so as to add or take away questions and options and magnificence the quiz nonetheless you need.
Now, whenever you run the equipment, you might select the options and submit the quiz to get the outcomes.
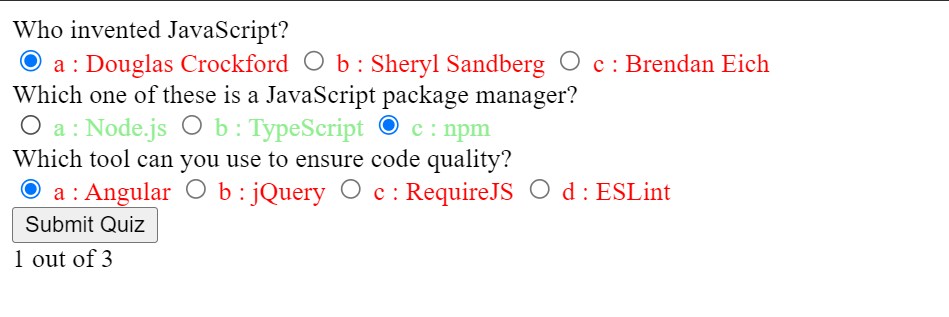
Step 5 – Together with Sorts
Since now now we have now a working quiz, let’s make it further shopper nice by together with some varieties. Nonetheless, I gained’t be going into particulars of each kind. You could straight copy the beneath code into the categories.css file.
@import url(https://fonts.googleapis.com/css?family=Work+Sans:300,600);
physique{
font-size: 20px;
font-family: 'Work Sans', sans-serif;
shade: #333;
font-weight: 300;
text-align: coronary heart;
background-color: #f8f6f0;
}
h1{
font-weight: 300;
margin: 0px;
padding: 10px;
font-size: 20px;
background-color: #444;
shade: #fff;
}
.question{
font-size: 30px;
margin-bottom: 10px;
}
.options {
margin-bottom: 20px;
text-align: left;
present: inline-block;
}
.options label{
present: block;
margin-bottom: 10px;
}
button{
font-family: 'Work Sans', sans-serif;
font-size: 22px;
background-color: #279;
shade: #fff;
border: 0px;
border-radius: 3px;
padding: 20px;
cursor: pointer;
margin-bottom: 20px;
}
button:hover{
background-color: #38a;
}
.slide{
place: absolute;
left: 0px;
excessive: 0px;
width: 100%;
z-index: 1;
opacity: 0;
transition: opacity 0.5s;
}
.active-slide{
opacity: 1;
z-index: 2;
}
.quiz-container{
place: relative;
prime: 200px;
margin-top: 40px;
}
At this degree, your quiz might appear as if this (with a tiny little little bit of styling):
As you may even see throughout the above footage, the questions throughout the quiz are ordered one after one different. We now must scroll down to select our options. Although this appears advantageous with three questions, you might start struggling to answer them when the number of questions will improve. So, we have now to find a way to current only one question at a time by means of pagination.
For that, you’ll need:
- A way to current and conceal questions.
- Buttons to navigate the quiz.
So, let’s make some modifications to our code, starting with HTML:
Most of that markup is equivalent as sooner than, nonetheless now we’ve added navigation buttons and a quiz container. The quiz container will help us place the questions as layers that we are going to current and conceal.
Subsequent, contained within the buildQuiz function, we have now so as to add a
output.push(
`
`
);
Subsequent, we’ll use some CSS positioning to make the slides sit as layers on excessive of one another. On this occasion, you’ll uncover we’re using z-indexes and opacity transitions to allow our slides to fade in and out. Proper right here’s what that CSS might appear as if:
.slide{
place: absolute;
left: 0px;
excessive: 0px;
width: 100%;
z-index: 1;
opacity: 0;
transition: opacity 0.5s;
}
.active-slide{
opacity: 1;
z-index: 2;
}
.quiz-container{
place: relative;
prime: 200px;
margin-top: 40px;
}
Now we’ll add some JavaScript to make the pagination work. As sooner than, order is important, so this the revised building of our code:
// Capabilities
// New capabilities go proper right here
// Variables
// Related code as sooner than
// Kick points off
buildQuiz();
// Pagination
// New code proper right here
// Current the first slide
showSlide(currentSlide);
// Event listeners
// New event listeners proper right here
We’re capable of start with some variables to retailer references to our navigation buttons and protect monitor of which slide we’re on. Add these after the choice to buildQuiz(), as confirmed above:
// Pagination
const previousButton = doc.getElementById("earlier");
const nextButton = doc.getElementById("subsequent");
const slides = doc.querySelectorAll(".slide");
let currentSlide = 0;
Subsequent we’ll write a function to point a slide. Add this beneath the current capabilities (buildQuiz and showResults):
function showSlide(n) {
slides[currentSlide].classList.take away('active-slide');
slides[n].classList.add('active-slide');
currentSlide = n;
if(currentSlide === 0){
previousButton.kind.present = 'none';
}
else{
previousButton.kind.present = 'inline-block';
}
if(currentSlide === slides.length-1){
nextButton.kind.present = 'none';
submitButton.kind.present = 'inline-block';
}
else{
nextButton.kind.present = 'inline-block';
submitButton.kind.present = 'none';
}
}
Proper right here’s what the first three strains do:
- Disguise the current slide by eradicating the active-slide class.
- Current the model new slide by together with the active-slide class.
- Exchange the current slide amount.
The next strains introduce the subsequent JavaScript logic:
- If we’re on the first slide, conceal the Earlier Slide button. In every other case, current the button.
- If we’re on the ultimate slide, conceal the Subsequent Slide button and current the Submit button. In every other case, current the Subsequent Slide button and conceal the Submit button.
After we’ve written our function, we’ll immediately identify showSlide(0) to point the first slide. This might come after the pagination code:
// Pagination
...
showSlide(currentSlide);
Subsequent we’ll write capabilities to make the navigation buttons work. These go beneath the showSlide function:
function showNextSlide() {
showSlide(currentSlide + 1);
}
function showPreviousSlide() {
showSlide(currentSlide - 1);
}
Proper right here, we’re making use of our showSlide function to allow our navigation buttons to point the sooner slide and the next slide.
Lastly, we’ll should hook the navigation buttons as a lot as these capabilities. This comes on the end of the code:
// Event listeners
...
previousButton.addEventListener("click on on", showPreviousSlide);
nextButton.addEventListener("click on on", showNextSlide);
Now your quiz has working navigation!
What’s Subsequent?
Now that you’ve a basic JavaScript quiz app, it’s time to get creative and experiment.
Listed beneath are some suggestions you might try:
- Try different methods of responding to an correct reply or a incorrect reply.
- Style the quiz correctly.
- Add a progress bar.
- Let prospects overview options sooner than submitting.
- Give prospects a summary of their options after they submit them.
- Exchange the navigation to let prospects skip to any question amount.
- Create personalized messages for each diploma of outcomes. As an example, if any person scores 8/10 or better, identify them a quiz ninja.
- Add a button to share outcomes on social media.
- Save your extreme scores using localStorage.
- Add a countdown timer to see if people can beat the clock.
- Apply the concepts from this textual content to completely different makes use of, paying homage to a mission worth estimator, or a social “which-character-are-you” quiz.
FAQs on Discover ways to Make a Straightforward JavaScript Quiz
How Can I Add Further Inquiries to the JavaScript Quiz?
Together with further inquiries to your JavaScript quiz is a straightforward course of. You will need to add further objects to the questions array in your JavaScript code. Each object represents a question and has two properties: textual content material (the question itself) and responses (an array of doable options). Proper right here’s an occasion of how one can add a model new question:
questions.push({
textual content material: 'What is the capital of France?',
responses: [
{
text: 'Paris',
correct: true
},
{
text: 'London',
correct: false
},
{
text: 'Berlin',
correct: false
},
{
text: 'Madrid',
correct: false
}
]
});
On this occasion, we’re together with a question regarding the capital of France, with 4 doable options. The correct reply is marked with ‘proper: true’.
How Can I Randomize the Order of Questions throughout the Quiz?
Randomizing the order of questions might make your quiz harder and pleasant. You could acquire this by using the sort() method blended with the Math.random() function. Proper right here’s how you’ll be able to do it:
questions.form(
function() {
return 0.5 - Math.random();
}
);
This code will randomly form the questions array each time the online web page is loaded.
How Can I Add a Timer to the Quiz?
Together with a timer might make your quiz further thrilling. You could merely add a timer to the quiz using the JavaScript setInterval() function. Proper right here’s a straightforward occasion:
var timeLeft = 30;
var timer = setInterval(function() {
timeLeft--;
doc.getElementById('timer').textContent = timeLeft;
if (timeLeft
On this occasion, the quiz will closing for 30 seconds. The timer will exchange every second, and when the time is up, an alert shall be confirmed.
How Can I Current the Acceptable Reply if the Client Will get it Mistaken?
You could current the right reply by modifying the checkAnswer() function. You could add an else clause to the if assertion that checks if the reply is correct. Proper right here’s how you’ll be able to do it:
function checkAnswer(question, response) {
if (question.responses[response].proper) {
ranking++;
} else {
alert('The correct reply is: ' + question.responses.uncover(r => r.proper).textual content material);
}
}
On this occasion, if the buyer’s reply is inaccurate, an alert shall be confirmed with the right reply.
How Can I Add Photographs to the Questions?
You could add footage to your questions by together with an ‘image’ property to the question objects. You could then use this property to indicate the image in your HTML. Proper right here’s an occasion:
questions.push({
textual content material: 'What's that this animal?',
image: 'elephant.jpg',
responses: [
{ text: 'Elephant', correct: true },
{ text: 'Lion', correct: false },
{ text: 'Tiger', correct: false },
{ text: 'Bear', correct: false }
]
});
In your HTML, you might present the image like this:
![]()
And in your JavaScript, you might exchange the src attribute of the image when displaying a model new question:
doc.getElementById('questionImage').src = question.image;
On this occasion, an image of an elephant shall be displayed when the question is confirmed.
How Do I Cope with Numerous Acceptable Options in a JavaScript Quiz?
Coping with quite a few proper options entails allowing the buyer to select a number of reply and checking if any of the chosen options are proper. As an example, proper right here is how one can exchange the above showResults() function to cope with quite a few proper options.
function showResults() {
const answerContainers = quizContainer.querySelectorAll('.options');
let numCorrect = 0;
myQuestions.forEach((currentQuestion, questionNumber) => {
const answerContainer = answerContainers[questionNumber];
const selector = `enter[name=question${questionNumber}]:checked`;
const userAnswers = Array.from(answerContainer.querySelectorAll(selector)).map(enter => enter.price);
if (userAnswers.form().toString() === currentQuestion.correctAnswers.form().toString()) {
numCorrect++;
answerContainers[questionNumber].kind.shade="lightgreen";
} else {
answerContainers[questionNumber].kind.shade="purple";
}
});
resultsContainer.innerHTML = `${numCorrect} out of ${myQuestions.measurement}`;
}
Is It Important to Protect Seperate JavaScript File and a CSS File?
Sustaining separate JavaScript and CSS recordsdata is not a ought to. Nonetheless, it is usually considered a most interesting observe as a result of it improves the readability and maintainability of your code.
Yaphi Berhanu is an web developer who loves serving to people improve their coding experience. He writes concepts and strategies at http://simplestepscode.com. In his totally unbiased opinion, he suggests checking it out.
Neighborhood admin, freelance web developer and editor at SitePoint.
CSS Animationsjameshmodernjs-tutorialsquiz