Correct proper right here’s how which can look:
This constructing is a simple event of uncover methods to create quiz HTML code that serves as a basis in your JavaScript quiz template. Inside the event you run the gear now, you might merely see a “Submit Quiz” button.
Step 2 – Initialize JavaScript Variables
Now, we’ll use the JavaScript doc.getElementById methodology to pick the above HTML components and retailer references to them all through the JavaScript quiz code like beneath:
const quizContainer = doc.getElementById('quiz');
const resultsContainer = doc.getElementById('outcomes');
const submitButton = doc.getElementById('submit');
The subsequent problem our quiz app wants is plenty of inquiries to point. We’ll use JavaScript object literals to signify the actual particular person questions and an array to carry all of the questions that make up our quiz app. Utilizing an array will make the questions straightforward to iterate over:
const myQuestions = [
{
question: "Who invented JavaScript?",
answers: {
a: "Douglas Crockford",
b: "Sheryl Sandberg",
c: "Brendan Eich"
},
correctAnswer: "c"
},
{
question: "Which one of these is a JavaScript package manager?",
answers: {
a: "Node.js",
b: "TypeScript",
c: "npm"
},
correctAnswer: "c"
},
{
question: "Which tool can you use to ensure code quality?",
answers: {
a: "Angular",
b: "jQuery",
c: "RequireJS",
d: "ESLint"
},
correctAnswer: "d"
}
];
Be fully glad to place in as many questions or choices as you need.
Observe: As that is an array, the questions will seem all through the order they’re listed. Within the occasion you wish to kind the questions in any approach prior to presenting them to the customer, try our fast tip on sorting an array of objects in JavaScript.
Step 3 – Assemble the Quiz Operate
Now that now we’ve now our doc of questions, we’ll present them on the web internet web page. For that, we will probably be utilizing a perform named buildQuix(). Let’s bear the following JavaScript line by line to see the easiest way it actually works:
perform buildQuiz(){
// variable to retailer the HTML output
const output = [];
// for every query...
myQuestions.forEach(
(currentQuestion, questionNumber) => {
// variable to retailer the doc of doable choices
const choices = [];
// and for every accessible reply...
for(letter in currentQuestion.choices){
// ...add an HTML radio button
choices.push(
``
);
}
// add this query and its choices to the output
output.push(
` ${currentQuestion.query}
${choices.be part of('')}
`
);
}
);
// lastly mix our output doc into one string of HTML and put it on the web internet web page
quizContainer.innerHTML = output.be part of('');
}
First, we create an output variable to comprise all of the HTML output, together with questions and reply picks.
Subsequent, we’ll begin establishing the HTML for every query. We’ll ought to loop by the use of every query like this:
myQuestions.forEach( (currentQuestion, questionNumber) => {
// the code we wish to run for every query goes correct proper right here
});
For brevity, we’re utilizing an arrow perform to carry out our operations on every query. On account of that is in a forEach loop, we get the present worth, the index (the place quantity of the present merchandise all through the array), and the array itself as parameters. We solely want the present worth and the index, which for our capabilities, we’ll decide currentQuestion and questionNumber respectively.
Now let’s have a look on the code inside our loop:
// we'll wish to retailer the doc of reply picks
const choices = [];
// and for every accessible reply...
for(letter in currentQuestion.choices){
// ...add an html radio button
choices.push(
``
);
}
// add this query and its choices to the output
output.push(
` ${currentQuestion.query}
${choices.be part of('')}
`
);
For every query, we’ll wish to generate the proper HTML. So, our first step is to create an array to carry the doc of doable choices.s.
Subsequent, we’ll use a loop to fill all through the doable choices for the present query. For every alternative, we’re creating an HTML radio button, which we enclose in a
Correct proper right here, we’re utilizing template literals, that are strings nonetheless additional extraordinarily environment friendly. We’ll make use of the following decisions of template literals:
- Multi-line capabilities.
- Don’t must make use of escape quotes inside quotes on account of template literals use backticks.
- String interpolation permits embedding JavaScript expressions appropriate into your strings like this: ${code_goes_here}.
As rapidly as now we’ve now our doc of reply buttons, we’ll push the query HTML and the reply HTML onto our complete doc of outputs.
Uncover that we’re utilizing a template literal and a few embedded expressions to first create the query div after which create the reply div. The be part of expression takes our doc of choices and areas them collectively in a single string that we’re going to output into our choices div.
Now that we’ve generated the HTML for every query, we will be part of all of it collectively and present it on the web internet web page:
quizContainer.innerHTML = output.be part of('');
Now, our buildQuiz perform is full, and it is best to have the ability to run the quiz app and see the questions displayed.
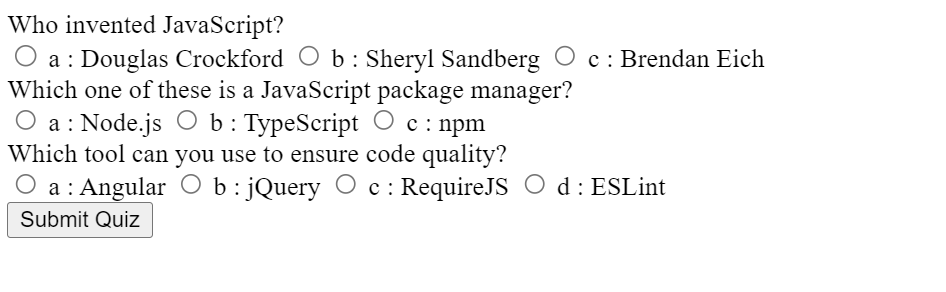
Nonetheless, the event of your code is necessary. On account of 1 issue often known as the temporal lifeless zone, you may’t reference your query array prior to it has been outlined.
To recap, that is the right constructing:
// Capabilities
perform buildQuiz(){ ... }
perform showResults(){ ... }
// Variables
const quizContainer = doc.getElementById('quiz');
const resultsContainer = doc.getElementById('outcomes');
const submitButton = doc.getElementById('submit');
const myQuestions = [ ... ];
// Kick factors off
buildQuiz();
// Occasion listeners
submitButton.addEventListener('click on on on', showResults);
Step 4 – Displaying the Quiz Outcomes
At this diploma, we wish to assemble out our showResults perform to loop over the choices, check out them, and present the outcomes. It is a necessary a part of any quiz recreation JavaScript implementation, because of it gives speedy suggestions to the customer based mostly completely on their effectivity.
Correct proper right here’s the perform, which we’ll bear intimately subsequent:
perform showResults(){
// accumulate reply containers from our quiz
const answerContainers = quizContainer.querySelectorAll('.choices');
// defend monitor of purchaser's choices
let numCorrect = 0;
// for every query...
myQuestions.forEach( (currentQuestion, questionNumber) => {
// uncover chosen reply
const answerContainer = answerContainers[questionNumber];
const selector = `enter[name=question${questionNumber}]:checked`;
const userAnswer = (answerContainer.querySelector(selector) || {}).worth;
// if reply is appropriate
if(userAnswer === currentQuestion.correctAnswer){
// add to the variety of correct choices
numCorrect++;
// shade the choices inexperienced
answerContainers[questionNumber].variety.shade="lightgreen";
}
// if reply is inaccurate or clear
else{
// shade the choices purple
answerContainers[questionNumber].variety.shade="purple";
}
});
// present variety of correct choices out of full
resultsContainer.innerHTML = `${numCorrect} out of ${myQuestions.measurement}`;
}
First, we choose all the reply containers in our quiz’s HTML. Then, we’ll create variables to maintain monitor of the customer’s present reply and the final variety of correct choices.
// accumulate reply containers from our quiz
const answerContainers = quizContainer.querySelectorAll('.choices');
// defend monitor of purchaser's choices
let numCorrect = 0;
Now, we’ll loop by the use of every query and check out the choices.
We will probably be utilizing 3 steps for that:
- Uncover the chosen reply all through the HTML.
- Deal with what occurs if the reply is appropriate.
- Deal with what occurs if the reply is inaccurate.
Let’s look additional fastidiously at how we’re discovering the chosen reply in our HTML:
// uncover chosen reply
const answerContainer = answerContainers[questionNumber];
const selector = `enter[name=question${questionNumber}]:checked`;
const userAnswer = (answerContainer.querySelector(selector) || {}).worth;
First, we’re guaranteeing we’re wanting contained within the reply container for the present query.
All through the following line, we’re defining a CSS selector which will allow us to search out which radio button is checked.
Then we’re utilizing JavaScript’s querySelector to hunt for our CSS selector all through the beforehand outlined answerContainer. In essence, which signifies that we’ll uncover which reply’s radio button is checked.
Lastly, we’ll get the worth of that reply by utilizing .worth.
Coping with Incomplete Shopper Enter
What if the customer has left a solution clear? On this case, utilizing .worth would set off an error on account of you may’t get the worth of 1 issue that’s not there. To resolve this, we’ve added ||, which suggests “or”, and {}, which is an empty object. Now, the general assertion says:
- Get a reference to our chosen reply half OR, if that doesn’t exist, use an empty object.
- Get the worth of no matter was all through the primary assertion.
In consequence, the worth will every be the customer’s reply or undefined, which suggests a client can skip a query with out crashing our quiz app.
Evaluating the Choices and Displaying the Consequence
The subsequent statements in our answer-checking loop will allow us to deal with correct and incorrect choices.
// if reply is appropriate
if(userAnswer === currentQuestion.correctAnswer){
// add to the variety of correct choices
numCorrect++;
// shade the choices inexperienced
answerContainers[questionNumber].variety.shade="lightgreen";
}
// if reply is inaccurate or clear
else{
// shade the choices purple
answerContainers[questionNumber].variety.shade="purple";
}
If the customer’s reply matches the proper alternative, improve the variety of correct choices by one and (optionally) shade the set of picks inexperienced. If the reply is inaccurate or clear, shade the reply picks purple (as quickly as further, elective).
As rapidly as a result of the answer-checking loop is completed, we’ll present what number of questions the customer bought appropriate:
// present variety of correct choices out of full
resultsContainer.innerHTML = `${numCorrect} out of ${myQuestions.measurement}`;
And now now we’ve now a working JavaScript quiz!
Inside the event you’d like, you may wrap all of the quiz in an IIFE (instantly invoked perform expression), which is a perform that runs as quickly as you outline it. This may occasionally more and more defend your variables out of world scope and make sure that your quiz app doesn’t intrude with one other scripts working on the web internet web page.
(perform(){
// put the remainder of your code correct proper right here
})();
Now you’re all set! Be fully glad so as in order so as to add or take away questions and choices and magnificence the quiz nonetheless you want.
Now, everytime you run the gear, you may choose the choices and submit the quiz to get the outcomes.
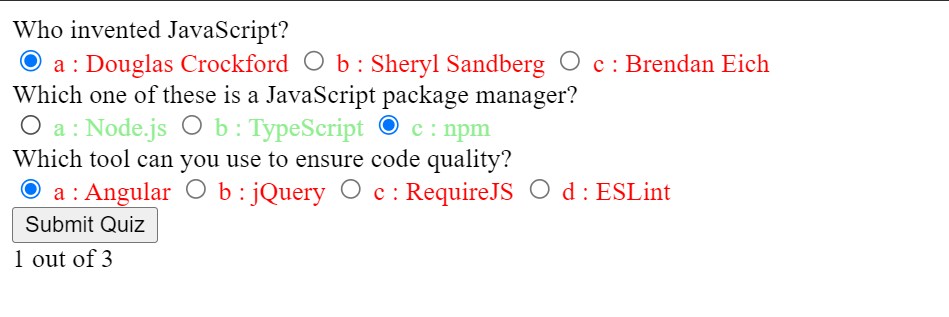
Step 5 – Along with Kinds
Since now now we’ve now a working quiz, let’s make it additional shopper good by along with some varieties. Nonetheless, I gained’t be going into particulars of every variety. You could possibly straight copy the beneath code into the classes.css file.
@import url(https://fonts.googleapis.com/css?household=Work+Sans:300,600);
physique{
font-size: 20px;
font-family: 'Work Sans', sans-serif;
shade: #333;
font-weight: 300;
text-align: coronary coronary heart;
background-color: #f8f6f0;
}
h1{
font-weight: 300;
margin: 0px;
padding: 10px;
font-size: 20px;
background-color: #444;
shade: #fff;
}
.query{
font-size: 30px;
margin-bottom: 10px;
}
.choices {
margin-bottom: 20px;
text-align: left;
current: inline-block;
}
.choices label{
current: block;
margin-bottom: 10px;
}
button{
font-family: 'Work Sans', sans-serif;
font-size: 22px;
background-color: #279;
shade: #fff;
border: 0px;
border-radius: 3px;
padding: 20px;
cursor: pointer;
margin-bottom: 20px;
}
button:hover{
background-color: #38a;
}
.slide{
place: absolute;
left: 0px;
extreme: 0px;
width: 100%;
z-index: 1;
opacity: 0;
transition: opacity 0.5s;
}
.active-slide{
opacity: 1;
z-index: 2;
}
.quiz-container{
place: relative;
prime: 200px;
margin-top: 40px;
}
At this diploma, your quiz may seem as if this (with a tiny little little little bit of styling):
As you might even see all through the above footage, the questions all through the quiz are ordered one after one totally different. We now should scroll down to pick our choices. Though this seems advantageous with three questions, you may begin struggling to reply them when the variety of questions will enhance. So, we’ve now to discover a strategy to present just one query at a time by the use of pagination.
For that, you’ll want:
- A strategy to present and conceal questions.
- Buttons to navigate the quiz.
So, let’s make some modifications to our code, beginning with HTML:
Most of that markup is equal as prior to, nonetheless now we’ve added navigation buttons and a quiz container. The quiz container will assist us place the questions as layers that we’re going to present and conceal.
Subsequent, contained inside the buildQuiz perform, we’ve now in order so as to add a
output.push(
`
`
);
Subsequent, we’ll use some CSS positioning to make the slides sit as layers on extreme of each other. On this event, you’ll uncover we’re utilizing z-indexes and opacity transitions to permit our slides to fade out and in. Correct proper right here’s what that CSS may seem as if:
.slide{
place: absolute;
left: 0px;
extreme: 0px;
width: 100%;
z-index: 1;
opacity: 0;
transition: opacity 0.5s;
}
.active-slide{
opacity: 1;
z-index: 2;
}
.quiz-container{
place: relative;
prime: 200px;
margin-top: 40px;
}
Now we’ll add some JavaScript to make the pagination work. As prior to, order is necessary, so this the revised constructing of our code:
// Capabilities
// New capabilities go correct proper right here
// Variables
// Associated code as prior to
// Kick factors off
buildQuiz();
// Pagination
// New code correct proper right here
// Present the primary slide
showSlide(currentSlide);
// Occasion listeners
// New occasion listeners correct proper right here
We’re able to begin with some variables to retailer references to our navigation buttons and defend monitor of which slide we’re on. Add these after the selection to buildQuiz(), as confirmed above:
// Pagination
const previousButton = doc.getElementById("earlier");
const nextButton = doc.getElementById("subsequent");
const slides = doc.querySelectorAll(".slide");
let currentSlide = 0;
Subsequent we’ll write a perform to level a slide. Add this beneath the present capabilities (buildQuiz and showResults):
perform showSlide(n) {
slides[currentSlide].classList.take away('active-slide');
slides[n].classList.add('active-slide');
currentSlide = n;
if(currentSlide === 0){
previousButton.variety.current = 'none';
}
else{
previousButton.variety.current = 'inline-block';
}
if(currentSlide === slides.length-1){
nextButton.variety.current = 'none';
submitButton.variety.current = 'inline-block';
}
else{
nextButton.variety.current = 'inline-block';
submitButton.variety.current = 'none';
}
}
Correct proper right here’s what the primary three strains do:
- Disguise the present slide by eradicating the active-slide class.
- Present the mannequin new slide by along with the active-slide class.
- Trade the present slide quantity.
The subsequent strains introduce the following JavaScript logic:
- If we’re on the primary slide, conceal the Earlier Slide button. In each different case, present the button.
- If we’re on the last word slide, conceal the Subsequent Slide button and present the Submit button. In each different case, present the Subsequent Slide button and conceal the Submit button.
After we’ve written our perform, we’ll instantly determine showSlide(0) to level the primary slide. This may come after the pagination code:
// Pagination
...
showSlide(currentSlide);
Subsequent we’ll write capabilities to make the navigation buttons work. These go beneath the showSlide perform:
perform showNextSlide() {
showSlide(currentSlide + 1);
}
perform showPreviousSlide() {
showSlide(currentSlide - 1);
}
Correct proper right here, we’re making use of our showSlide perform to permit our navigation buttons to level the earlier slide and the subsequent slide.
Lastly, we’ll ought to hook the navigation buttons as quite a bit as these capabilities. This comes on the top of the code:
// Occasion listeners
...
previousButton.addEventListener("click on on on", showPreviousSlide);
nextButton.addEventListener("click on on on", showNextSlide);
Now your quiz has working navigation!
What’s Subsequent?
Now that you’ve got a primary JavaScript quiz app, it’s time to get inventive and experiment.
Listed beneath are some strategies you may strive:
- Strive totally different strategies of responding to an appropriate reply or a incorrect reply.
- Type the quiz appropriately.
- Add a progress bar.
- Let prospects overview choices prior to submitting.
- Give prospects a abstract of their choices after they submit them.
- Trade the navigation to let prospects skip to any query quantity.
- Create customized messages for every diploma of outcomes. For example, if any particular person scores 8/10 or higher, determine them a quiz ninja.
- Add a button to share outcomes on social media.
- Save your excessive scores utilizing localStorage.
- Add a countdown timer to see if individuals can beat the clock.
- Apply the ideas from this textual content material to fully totally different makes use of, paying homage to a mission price estimator, or a social “which-character-are-you” quiz.
FAQs on Uncover methods to Make a Easy JavaScript Quiz
How Can I Add Additional Inquiries to the JavaScript Quiz?
Along with additional inquiries to your JavaScript quiz is a simple course of. You will have so as to add additional objects to the questions array in your JavaScript code. Every object represents a query and has two properties: textual content material materials (the query itself) and responses (an array of doable choices). Correct proper right here’s an event of how one can add a mannequin new query:
questions.push({
textual content material materials: 'What's the capital of France?',
responses: [
{
text: 'Paris',
correct: true
},
{
text: 'London',
correct: false
},
{
text: 'Berlin',
correct: false
},
{
text: 'Madrid',
correct: false
}
]
});
On this event, we’re along with a query relating to the capital of France, with 4 doable choices. The right reply is marked with ‘correct: true’.
How Can I Randomize the Order of Questions all through the Quiz?
Randomizing the order of questions may make your quiz tougher and nice. You could possibly purchase this by utilizing the type() methodology blended with the Math.random() perform. Correct proper right here’s how you can do it:
questions.kind(
perform() {
return 0.5 - Math.random();
}
);
This code will randomly kind the questions array every time the web internet web page is loaded.
How Can I Add a Timer to the Quiz?
Along with a timer may make your quiz additional thrilling. You could possibly merely add a timer to the quiz utilizing the JavaScript setInterval() perform. Correct proper right here’s an easy event:
var timeLeft = 30;
var timer = setInterval(perform() {
timeLeft--;
doc.getElementById('timer').textContent = timeLeft;
if (timeLeft
On this event, the quiz will closing for 30 seconds. The timer will alternate each second, and when the time is up, an alert shall be confirmed.
How Can I Present the Acceptable Reply if the Shopper Will get it Mistaken?
You could possibly present the proper reply by modifying the checkAnswer() perform. You could possibly add an else clause to the if assertion that checks if the reply is appropriate. Correct proper right here’s how you can do it:
perform checkAnswer(query, response) {
if (query.responses[response].correct) {
rating++;
} else {
alert('The right reply is: ' + query.responses.uncover(r => r.correct).textual content material materials);
}
}
On this event, if the customer’s reply is inaccurate, an alert shall be confirmed with the proper reply.
How Can I Add Pictures to the Questions?
You could possibly add footage to your questions by along with an ‘picture’ property to the query objects. You could possibly then use this property to point the picture in your HTML. Correct proper right here’s an event:
questions.push({
textual content material materials: 'What's that this animal?',
picture: 'elephant.jpg',
responses: [
{ text: 'Elephant', correct: true },
{ text: 'Lion', correct: false },
{ text: 'Tiger', correct: false },
{ text: 'Bear', correct: false }
]
});
In your HTML, you may current the picture like this:
![]()
And in your JavaScript, you may alternate the src attribute of the picture when displaying a mannequin new query:
doc.getElementById('questionImage').src = query.picture;
On this event, a picture of an elephant shall be displayed when the query is confirmed.
How Do I Deal with Quite a few Acceptable Choices in a JavaScript Quiz?
Dealing with fairly a number of correct choices entails permitting the customer to pick plenty of reply and checking if any of the chosen choices are correct. For example, correct proper right here is how one can alternate the above showResults() perform to deal with fairly a number of correct choices.
perform showResults() {
const answerContainers = quizContainer.querySelectorAll('.choices');
let numCorrect = 0;
myQuestions.forEach((currentQuestion, questionNumber) => {
const answerContainer = answerContainers[questionNumber];
const selector = `enter[name=question${questionNumber}]:checked`;
const userAnswers = Array.from(answerContainer.querySelectorAll(selector)).map(enter => enter.worth);
if (userAnswers.kind().toString() === currentQuestion.correctAnswers.kind().toString()) {
numCorrect++;
answerContainers[questionNumber].variety.shade="lightgreen";
} else {
answerContainers[questionNumber].variety.shade="purple";
}
});
resultsContainer.innerHTML = `${numCorrect} out of ${myQuestions.measurement}`;
}
Is It Necessary to Defend Seperate JavaScript File and a CSS File?
Sustaining separate JavaScript and CSS recordsdata shouldn’t be a must. Nonetheless, it’s normally thought-about a most attention-grabbing observe because of it improves the readability and maintainability of your code.
Yaphi Berhanu is an internet developer who loves serving to individuals enhance their coding expertise. He writes ideas and methods at http://simplestepscode.com. In his completely unbiased opinion, he suggests checking it out.
Neighborhood admin, freelance internet developer and editor at SitePoint.
CSS Animationsjameshmodernjs-tutorialsquiz